JAVA EXERCISES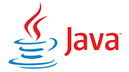
Requirements, Source & Output
[Java Interview Material]
REQUIREMENT
How to calculate the number of vowels and consonants in a string?
SOURCE
String text = "I am showing some some string.";
text = text.toLowerCase();
int vowels = 0;
int consonants = 0;
for (int i = 0; i < text.length(); i++) {
char c = text.charAt(i);
if (!(Character.isAlphabetic(c))) continue;
if (c == 'a' || c == 'e' || c == 'i' ||
c == 'o' || c == 'u')
vowels ++;
else
consonants ++;
}
out.println("Vowel count is: " + vowels);
out.println("Consonant count is: " + consonants);
OUTPUT
Vowel count is: 7
Consonant count is: 13
REQUIREMENT
How to calculate the number of numerical digits in a string?
SOURCE
String str = "TEST33u45644";
str = str.trim();
int len1 = str.length();
str=str.replaceAll("\\d", "");
int l = str.length();
out.println("The length of the string originally typed was: " + (len1));
out.println("The number of digits is: " + (len1-l));
OUTPUT
The length of the string originally typed was: 12
The number of digits is: 7
REQUIREMENT
How to count the occurrence of a particular character in a string?
SOURCE
String str2 = "TEST TEST 123 TEST";
char ch = 'E';
int cnt = 0;
char[] chararray = str2.toCharArray();
for (int i=0; i< chararray.length; i++){
if (chararray[i] == ch) cnt++;
}
out.println("Number of occurrences of "+ch+": " +cnt);
OUTPUT
Number of occurrences of E: 3
REQUIREMENT
Determine the factors of a number.
SOURCE
int num = 1024;
out.println("Factors for "+num+" are: ");
for (int i = 0; i< num; i++){
if(num%i==0) out.println(i +" ");
}
OUTPUT
Factors for 1024 are:
1
2
4
8
16
32
64
128
256
512
REQUIREMENT
How to perform swapping two strings by not using a third variable?
SOURCE
String str_1 = "String_1";
String str_2 = "String_2";
str_2 = str_1.concat(str_2);
str_1 = str_2.substring(str_1.length(), str_2.length());
str_2 = str_2.substring(0, str_1.length());
out.println("RESULT: str_1 is now: "+str_1+". str_2 is now: "+str_2 +".");
OUTPUT
RESULT: str_1 is now: String_2. str_2 is now: String_1.
REQUIREMENT
Determine if two strings are an anagram. [Def. Anagram: a word formed by rearranging the letters of another, such as cinema, formed from iceman.]
SOURCE
boolean is_anagram = false;
String str__1 = "cinema";
String str__2 = "iceman";
if( str__2.trim().length() != str__1.trim().length()) is_anagram=false;
else{
char[] str1chars = str__1.toCharArray();
char[] str2chars = str__2.toCharArray();
Arrays.sort(str1chars);
Arrays.sort(str2chars);
if (Arrays.equals(str1chars, str2chars)){
is_anagram=true;
}
}
out.println("RESULT: Are the two strings anagrams? "+is_anagram);
OUTPUT
RESULT: Are the two strings anagrams? true
REQUIREMENT
Determine if a number is prime or not.
SOURCE
boolean is_prime=false;
int numtotest = 11;
if (numtotest<=1) is_prime=false;
else{
for (int i=2; i< numtotest/2; i++){
if((numtotest%i)==0) {
is_prime=false;
break;
}
is_prime=true;
}
}
out.println("Is "+numtotest+" a prime number? RESULT: "+is_prime);
OUTPUT
Is 11 a prime number? RESULT: true
REQUIREMENT
List the first 10 numbers of the fibonacci sequence. [Fibonacci Sequence is where next number is found by adding up the two numbers before it.]
SOURCE
int endnumber = 10;
int firstnumber = 0;
int secondnumber = 1;
out.print("Fibonacci sequence up to "+endnumber+" results. RESULT: ");
for (int i=1; i <= endnumber; i++){
out.print(firstnumber+" ");
int nextnumber = firstnumber+secondnumber;
firstnumber = secondnumber;
secondnumber = nextnumber;
}
OUTPUT
Fibonacci sequence up to 10 results. RESULT: 0 1 1 2 3 5 8 13 21 34
REQUIREMENT
Remove all whitespace from a string.
SOURCE
String thisstring = "This is a sample string !";
thisstring = thisstring.replaceAll(" ", "");
out.println("String with no spaces: "+thisstring);
OUTPUT
String with no spaces: Thisisasamplestring!
REQUIREMENT
Find the second largest number in an array.
SOURCE
int arr[] = { 100 , 33, 46, 47, 122, 94, 52, 150, 36, 94, 89 };
int largest = 0;
int secondLargest = 0;
out.println("The given array is:");
for (int i : arr) //advanced for loop
{
out.print(i+" ");
}
out.println("");
for (int i = 0; i < arr.length; i++) //regular for loop
{
if (arr[i] > largest)
{
secondLargest = largest;
largest = arr[i];
}
else if (arr[i] > secondLargest)
{
secondLargest = arr[i];
}
}
out.println("The second largest number is: " + secondLargest);
OUTPUT
The given array is:
100 33 46 47 122 94 52 150 36 94 89
The second largest number is: 122
REQUIREMENT
Write a Java Program to find whether a string is palindrome or not. [Palindrome: A string that spells same word forward and reverse i.e. "dad" ]
SOURCE
String origstr = "dad";
String reversestr = "";
for (int i=origstr.length()-1; i>=0; i--){
reversestr = reversestr +origstr.charAt(i);
}
out.println("Orig String is: "+origstr);
out.println("Reverse String is: "+reversestr);
out.println("Palindrome?: "+origstr.equals(reversestr));
OUTPUT
Orig String is: dad
Reverse String is: dad
Palindrome?: true
REQUIREMENT
Write a Java Program to count the number of words in a string using HashMap.
SOURCE
String sentance1 = "This is a long string that says helloworld. Thank you.";
HashMap< Integer, String> words_hm= new HashMap();
String[] words = sentance1.split(" ");
for (int i=0; i< words.length; i++){
words_hm.put(i, words[i]);
}
out.println("Word count: "+ words_hm);
OUTPUT
Word count: {0=This, 1=is, 2=a, 3=long, 4=string, 5=that, 6=has, 7=multiple, 8=words, 9=helloworld., 10=, 11=Thank, 12=you.}
Java Language - Framework & Design
JVM, JDK & JRE - What are they?
[https://www.ibm.com/cloud/blog/jvm-vs-jre-vs-jdk]
What is Java Virtual Machine (JVM)?
Java Virtual Machine, or JVM, loads, verifies and executes Java bytecode. It is known as the interpreter or the core of Java programming language because it executes Java programming.
The role of JVM in Java
JVM is specifically responsible for converting bytecode to machine-specific code and is necessary in both JDK and JRE. It is also platform-dependent and performs many functions, including memory management and security. In addition, JVM can run programs written in other programming languages that have been translated to Java bytecode.
Java Native Interface (JNI) is often referred to in connection with JVM. JNI is a programming framework that enables Java code running in JVM to communicate with (i.e., to call and be called by) applications associated with a piece of hardware and specific operating system platform. These applications are called native applications and can often be written in other languages. Native methods are used to move native code written in other languages into a Java application.
JVM components
JVM consists of three main components or subsystems:
- Class Loader Subsystem is responsible for loading, linking and initializing a Java class file (i.e., “Java file”), otherwise known as dynamic class loading.
- Runtime Data Areas contain method areas, PC registers, stack areas and threads.
- Execution Engine contains an interpreter, compiler and garbage collection area.
What is Java Runtime Environment (JRE)?
Java Runtime Environment, or JRE, is a set of software tools responsible for execution of the Java program or application on your system.
JRE uses heap space for dynamic memory allocation for Java objects. JRE is also used in JDB (Java Debugging).
The role of JRE in Java
If a programmer would like to execute a Java program using the Java command, they should install JRE. If they are only installing (and not developing or compiling code), then only JRE is needed.
JRE components
Besides the Java Virtual Machine, JRE is composed of a variety of other supporting software tools and features to get the most out of your Java applications.
- Deployment solutions: Included as part of the JRE installation are deployment technologies like Java Web Start and Java Plugin that simplify the activation of applications and provide advanced support for future Java updates.
- Development toolkits: JRE also contains development tools designed to help developers improve their user interface. Some of these toolkits include the following:
- Java 2D: An application programming interface (API) used for drawing two-dimensional graphics in Java language. Developers can create rich user interfaces, special effects, games and animations.
- Abstract Window Toolkit (AWT): A Graphical User Interface (GUI) used to create objects, buttons, scroll bars and windows.
- Swing: Another lightweight GUI that uses a rich set of widgets to offer flexible, user-friendly customizations.
- Integration libraries: Java Runtime Environment provides several integration libraries and class libraries to assist developers in creating seamless data connections between their applications and services. Some of these libraries include the following:
- Java IDL: Uses Common Object Request Brokerage Architecture (CORBA) to support distributed objects written in Java programming language.
- Java Database Connectivity (JDBC) API: Provides tools for developers to write applications with access to remote relationship databases, flat files and spreadsheets.
- Java Naming and Directory Interface (JNDI): A programming interface and directory service that lets clients create portable applications that can fetch information from databases using naming conventions.
- Language and utility libraries: Included with JRE are Java.lang. and Java.util. packages that are fundamental for the design of Java applications, package versioning, management and monitoring. Some of these packages include the following
- Collections framework: A unified architecture made up of a collection of interfaces designed to improve the storage and process of application data.
- Concurrency utilities: A powerful framework package with high-performance threading utilities.
- Preferences API: A lightweight, cross-platform persistent API that enables multiple users on the same machine to define their own group of application preferences.
- Logging: Produces log reports — such as security failures, configuration errors, and performance issues — for further analysis.
- Java Archive (JAR): A platform-independent file format that enables multiple files to be bundled in JAR file format, significantly improving download speed and reducing file size.
What is Java Development Kit (JDK)?
Java Development Kit, or JDK, is a software development kit often called a superset of JRE. It is the foundational component that enables Java application and Java applet development. It is platform-specific, so separate installers are needed for each operating system (e.g., Mac, Unix and Windows).
The role of JDK in Java
JDK contains all the tools required to compile, debug and run a program developed using the Java platform. (It’s worth noting that Java programs can also be run using command line.)
JDK components
JDK includes all the Java tools, executables and binaries needed to run Java programs. This includes JRE, a compiler, a debugger, an archiver and other tools that are used in Java development.
Java SE vs. Java EE
Java is synonymous with Java Standard Edition (Java SE) or Core Java. All three euphemisms refer to the basic Java specification that includes the act of defining types and objects. Java EE, on the other hand, provides APIs and is typically used to run larger applications. The content of this blog focuses on Java SE.
How JVM, JRE and JDK work together
Let’s first look at how the three core components of Java work together, and then we can examine the differences. The diagram below provides an image of how JVM, JRE and JDK fit together in the Java landscape.
If you envision a baseball sliced open, it contains three main components: the round cushioned core, the wool and cotton midsection and the cowhide exterior. A ball without all three of these layers will not perform its intended function. Much like the three basic parts of a baseball, JVM, JRE and JDK all have very specific functions. Without all three, Java will not operate successfully.
If you envision a baseball sliced open, it contains three main components: the round cushioned core, the wool and cotton midsection and the cowhide exterior. A ball without all three of these layers will not perform its intended function. Much like the three basic parts of a baseball, JVM, JRE and JDK all have very specific functions.
JDK vs. JRE vs. JVM: Key differences
And now, for the differences:
- JDK is the development platform, while JRE is for execution.
- JVM is the foundation, or the heart of Java programming language, and ensures the program’s Java source code will be platform-agnostic.
- JVM is included in both JDK and JRE – Java programs won’t run without it.
Complementary technologies
There are many complementary technologies that can be used to enhance JVM, JRE or JDK. The following technologies are among the most frequently used:
- Just-in-time Compiler (JIT) is part of JVM and optimizes the conversion of bytecode to machine code. It selects similar bytecodes to compile at the same time, reducing the overall duration of bytecode to machine code compilation.
- Javac, another complementary tool, is a compiler that reads Java definitions and translates them into bytecode that can run on JVM.
- Javadoc converts API documentation from Java source code to HTML. This is useful when creating standard documentation in HTML.
OO Design in Java?
[https://hackr.io/blog/oops-concepts-in-java-with-examples]
OOPS Concepts in Java
Object-oriented programming is an approach to development and an organization that attempts to eliminate some of the flaws of conventional programming methods by incorporating the best of structured programming features with several new concepts. It involves new ways of organizing and developing programs and does not a concern using a particular language. Languages supporting OOP features include Smalltalk, Objective C, C++, Ada, Pascal, and Java.
Definition of OOPS Concepts in Java
So we can define OOP programming as:
“Object-oriented programming is an approach that modularizes programs by creating a partitioned memory area for both functions and data that can be used as templates for creating copies of such modules on demand.”
OOPS Paradigm
The primary objective of the object-oriented approach is to eliminate some of the pitfalls that exist in the procedural approach. OOP treats data as an element in the program, not allowing it to flow around the system freely. It ties data closely to the functions that operate on it and protects it from unintentional modification by other existing functions. OOPS allows decomposing a problem into several entities called Objects and then build data and functions from these entities. The combination of the data makes up an object.
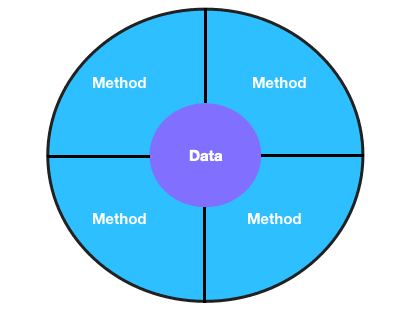
338.7K
Android Development Course For Beginners
Object = Method + Data
The data of an object is accessed by the methods associated with that object. However, the methods of an object can access methods of other objects.
Features of OOPS
Some features of object-oriented programming in java are:
- Emphasis is on data than procedures
- Programs are divided into objects
- Data Structures are designed to characterize objects.
- Methods operating on the data of an object are tied together in the data structure.
- Data is hidden, and external functions cannot access it.
- Objects communicate with each other through methods
- New methods and data can be easily added whenever necessary
- Follows the bottom-up approach in program design
List of OOPS Concepts in Java with Examples
General OOPS concepts in Java are:
Objects and Classes
Objects are runtime entities in an object-oriented system. An object can represent a person, a bank account, a place, a table of data. It may also represent user-defined data types like lists and vectors. Any programming problem is analyzed based on objects and how they communicate amongst themselves. The objects interact with each other by sending messages to one another when a program is executed. For Example, ‘customer’ and ‘account’ are two objects that may send a message to the account object requesting for the balance. Each object contains code and data to manipulate the data. Objects can even interact without knowing the details of each other’s code or data.
The entire set of code and data of an object can be made user-defined data type using the concept of the class. A class is a ‘data-type’ and an object as a ‘variable’ of that type. Any number of objects can be created after a class is created.
The collection of objects of similar types is termed as a class. For Example, apple, orange, and mango are the objects of the class Fruit. Classes behave like built-in data types of a programming language but are user-defined data types.
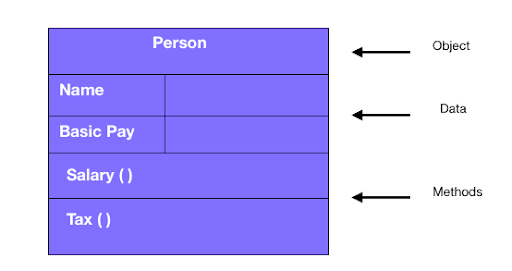
Representation of an Object
Data Abstraction and Encapsulation
The wrapping up of the data and methods into the single unit is known as encapsulation. The data is accessible only to those methods, which are wrapped in the class, and not to the outside world. This insulation of data from the direct access of the program is called data hiding. Encapsulation of the object makes it possible for the objects to be treated like ‘black boxes’ that perform a specific task without any concern for internal implementation.
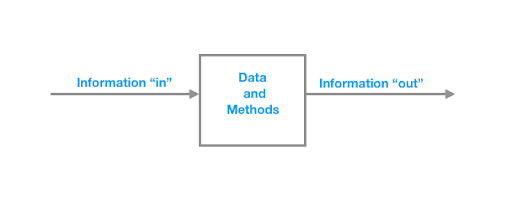
Encapsulation- Objects as “black-boxes”
Abstraction is the act of reducing programming complexity by representing essential features without including the background explanations or details. Classes are the concept of abstraction and are defined as the list of abstract attributes such as size, weight, cost, and methods that operate on these attributes. Classes wrap or encapsulate all the essential properties of the objects that are to be created.
Abstract classes and Abstract methods:
- An abstract class is a class with an abstract keyword.
- An abstract method is a method declared without a method body.
- An abstract class may not have all the abstract methods. Some methods are concrete.
- A method defined abstract must have its implementation in the derived class, thus making method overriding compulsory. Making a subclass abstract avoids overriding.
- Classes that contain abstract method(s) must be declared with abstract keyword.
- The object for an abstract class cannot be instantiated with the new operator.
- An abstract class can have parameterized constructors.
Ways to achieve abstraction:
- Using abstract keyword
- Using interfaces
Example Code:
The code below shows an example of abstraction.
abstract class Car
{
Car()
{
System.out.println("Car is built. ");
}
abstract void drive();
void gearChange()
{
System.out.println("Gearchanged!!");
}
}
class Tesla extends Car
{
void drive()
{
System.out.println("Drive Safely");
}
}
class Abstraction
{
public static void main (String args[])
{
Car obj = new Tesla();
obj.drive();
obj. gearChange();
}
}
Inheritance
Inheritance is the process by which objects of one class acquire some properties of objects of another class. Inheritance supports the concept of hierarchical classification. For Example, a bird Robin is part of the class, not a mammal, which is again a part of the class Animal. The principle behind this division is that each subclass shares common characteristics from the class from its parent class.
Properties of Inheritance
In OOP, the idea of inheritance provides the concept of reusability. It means that we can add additional features to parent class without modification; this is possible by deriving a new class from the parent class. The new class consists of the combined features from both the classes. In Java, the derived class is also known as the subclass.
Types of Inheritance
- Single
- Multiple
- Multilevel
- Hybrid
Example Code:
The code below illustrates an example of Inheritance.
class Animal
{
void eat()
{
System.out.println("I am a omnivorous!! ");
}
}
class Mammal extends Animal
{
void nature()
{
System.out.println("I am a mammal!! ");
}
}
class Dog extends Mammal
{
void sound()
{
System.out.println("I bark!! ");
}
}
class Inheritance
{
public static void main(String args[])
{
Dog d = new Dog();
d.eat();
d.nature();
d.sound();
}
}
Polymorphism
Polymorphism is an important OOP concept; it means the ability to take many forms. For Example, an operation exhibits different behavior in different situations. The behavior depends on the type of data used in operation. For Example, in the operation of addition, the operation generates a sum for two numbers. If the operands are strings, then a third-string is produced by the operation by concatenation.
The figure below demonstrates that a single function name can be used to handle the different numbers and different types of arguments.
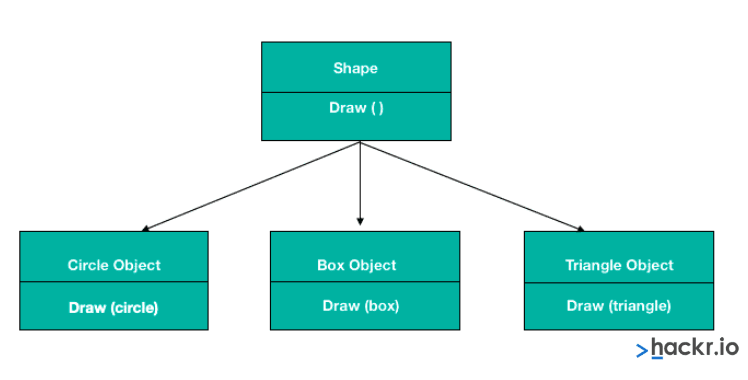
In polymorphism, objects having different internal structures can share the same external interface; it means that a class of operation may be accessed in the same manner even though actions with each operation may differ. Inheritance extensively uses the concept of polymorphism.
Polymorphism can be achieved in two ways:
Method Overloading
It is possible to create methods with the same name but different parameter lists and different definitions. This is called method overloading. Method overloading is required when objects are required to perform similar tasks but using different input parameters. When we call a method in an object, Java matches up the method name first and then the number and type of parameters to decide which definition to execute.
Method overloading is achieved in three ways:
On the Basis of | Example |
Number of Parameters | times(int, int) times(int, int, int) |
Data Types of Parameters | times(int, int) times(int, float) |
The Sequence of Data Types of Parameters | times(int, float) times(float, int) |
Example Code:
The code below demonstrates the concept of method overloading.
class CircleArea
{
double area(double x)
{
return 3.14 * x;
}
}
class SquareArea
{
int area(int x)
{
return x * x;
}
}
class RectangleArea
{
int area(int x, int y)
{
return x * y;
}
}
class TriangleArea
{
int area(int y, int x)
{
return (y * x)/2;
}
}
class Overloading
{
public static void main(String args[])
{
CircleArea ca = new CircleArea();
SquareArea sa = new SquareArea();
RectangleArea ra = new RectangleArea();
TriangleArea ta = new TriangleArea();
System.out.println("Circle area = "+ ca.area(1));
System.out.println("Square area = "+ sa.area(2));
System.out.println("Rectangle area = "+ ra.area(3,4));
System.out.println("Triangle area = "+ ta.area(6,3));
}
}
Method Overriding
A method defined in the superclass is inherited by its subclass and is used by the objects created by the subclass. However, there may be occasions when an object should respond to the same method but behave differently when that method is called, which means a method defined in the superclass is overridden. Overriding is achieved by defining a method in the subclass that has the same name, the same arguments, and the same return type as a method in the superclass. So, when the method is called, the method defined in the subclass invoked and executed instead of the one in the superclass.
Example Code:
The code below demonstrates the concept of method overriding.
class Shape
{
void draw()
{
System.out.println("Mention shape here");
}
void numberOfSides()
{
System.out.println("side = 0");
}
}
class Circle extends Shape
{
void draw()
{
System.out.println("CIRCLE ");
}
void numberOfSides()
{
System.out.println("side = 0 ");
}
}
class Box extends Shape
{
void draw()
{
System.out.println("BOX ");
}
void numberOfSides()
{
System.out.println("side= 6");
}
}
class Triangle extends Shape
{
void draw()
{
System.out.println("TRIANGLE ");
}
void numberOfSides()
{
System.out.println("side = 3 ");
}
}
class Overriding
{
public static void main (String args[])
{
Circle c = new Circle();
c.draw();
c.numberOfSides();
Box b = new Box();
b.draw();
b.numberOfSides();
Triangle t = new Triangle();
t.draw();
t.numberOfSides();
}
}
Dynamic Binding
Binding is the process of linking a procedure call to the code to be executed in response to the call. It means that the code associated with the given procedure call is not known until the time of the call at runtime. It is associated with inheritance and polymorphism.
Message Communication
Objects communicate with each other in OOPs The process of programming in case of OOP consists of the following:
- Creating classes defining objects and their behavior.
- Creating objects
- Establishing communication between objects.
The network of Objects Communicating with Each Other
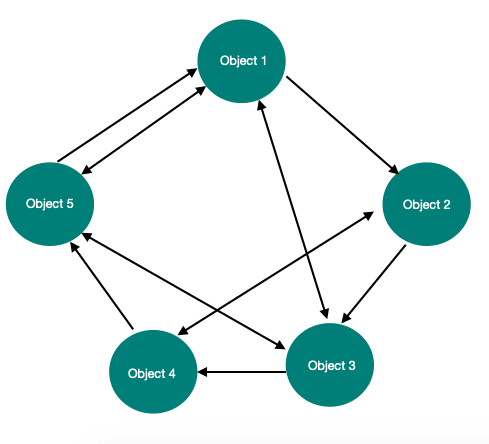
Specifying the object name, the method name, and the information to be sent is involved in message passing.
For Example, consider the statement.
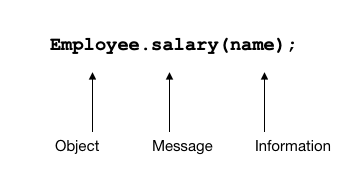
Objects can be created or destroyed as they have a life cycle. It allows communication between the objects until the objects are alive.
Benefits of OOPs Concept in Java
- Inheritance eliminates redundant code and enables reusability.
- As Message passing allows communication with objects, this presents writing code from scratch every time. It is thus saving development time and higher productivity.
- Partitions work in a project based on classes and objects.
- Systems up-gradation is easy.
Applications of OOPs Concept in Java
- Real-time systems
- Simulation and modeling
- Object-oriented databases
- Hypertext and Hypermedia
- AI and expert systems
- Neural networks and parallel programming
- Automation systems
Summary
Java is a robust and scalable object-oriented programming language that is based on the concept of objects and classes. It offers features like inheritance, abstraction, encapsulation, and polymorphism for developing an efficient and reliable code.
People are also reading: